JavaScript ES6 introduced two new data structures – maps and sets. These works similar to objects and arrays. Let’s see how to create a map data structure.
Map Data structure
A map is an ordered collection of key-value pairs. Maps are like objects. However, unlike objects maps are ordered and their keys need not be of string data type. They can be of any data type. Since it is ordered they can be iterable like arrays. Let’s see the syntax to create a map data structure.
Syntax:
let map = new Map([iterable])
let map = new Map()
Properties:
Map.prototype.size – returns the number of key-value pairs in Map object
Syntax:
Map.size
Methods:
- set() – add/update a key value entry
- get() – returns a value mapped to a specified key
- has() – returns a Boolean indicating if a specific key exists or not
- forEach() – executes a provided function for each key value pair
- keys() – returns a new iterator object that contains the key of each element
- values() – returns a new iterator object that contains the value of each element
- entries() – returns a new iterator object that contains the [key, value] pairs of each element
- delete() – removes specified elements from object map
- clear() – removes all elements from object map
Now let us see some of the above methods properties with example.
let emp = new Map();
//set
emp.set('Name','John');
emp.set('ID','101');
emp.set('Salary','10000');
//get
emp.get('Name');
//size
console.log(emp.size);
//has
console.log(emp.has('ID'));
//keys
for (let key of emp.keys()) {
console.log(key);
}
//values
for (let value of emp.values()) {
console.log(value);
}
//entries
for (let entry of emp.entries()) {
console.log(entry);
}
//delete
console.log(emp.delete('Salary'));
console.log(emp.has('Salary'));
//clear
console.log(emp.size)
emp.clear();
console.log(emp.size)
Output:
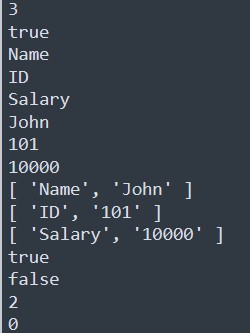
How to convert object to map?
To create a map from object we can convert the object into an array of [key, value] pairs and then create a map using it.
Object.entries() – used to convert object to map.
let obj = {
"Name" : "John",
"ID" : "101";
"Salary" : "10000"
}
let newarr = Object.entries(obj);
let map = new Map(newarr);
console.log(map);

How to convert map to object?
Here we use object.fromEntries() to convert a map to object.
const map = new Map([['Name','John'],['ID','101'],['Salary','10000']]);
consr obj = Object.fromEntries(map);
console.log(obj);
Output:

To know more about javascript data structures click here!