CRUD operations in SQL
CRUD is an acronym that stands for Create, Read, Update and Delete.
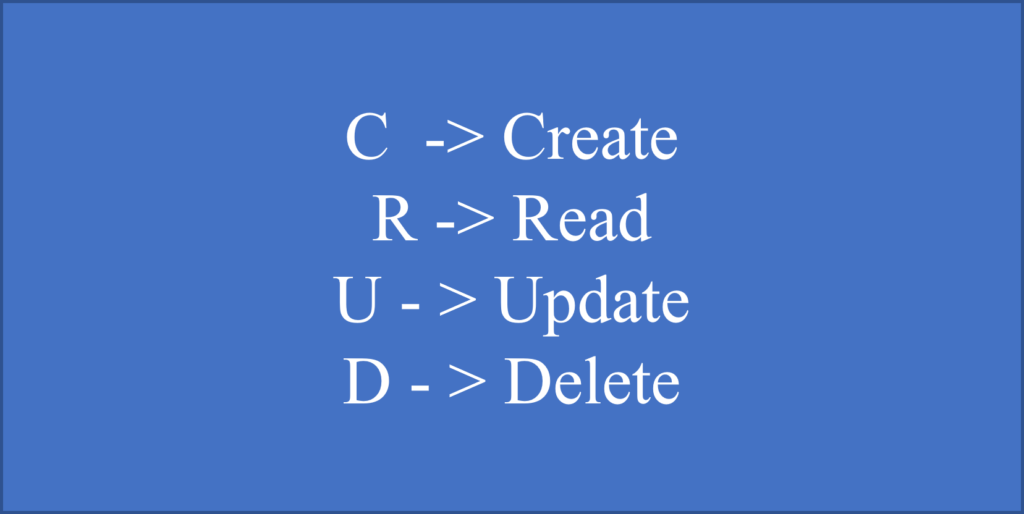
Each letter in acronym refers to all functions executed in a database and mapped to standard HTTP method, SQL statement. CRUD is data-oriented and standardized use of HTTP action methods. This consists of four basic operations which we can perform in a Database table.
Each letter in CRUD corresponds to HTTP request method.
- Create –> POST
- Read –> GET
- Update –> PUT or PATCH
- Delete –> DELETE
Create:
Create means to add or insert data into the table. First we will create table using CREATE TABLE command and then add data using INSERT INTO command.
SQL Command to create table:
CREATE TABLE employee(EmpID int PRIMARY KEY, FIRST_NAME VARCHAR, Salary INT);
SQL command to insert data:
INSERT INTO employee(EmpID, FIRST_NAME, Salary) VALUES (1, ‘Anna’, 10000), (2, ‘Maria’, 20000), (3, ‘Thomas’, 30000);
EmpID | FIRST_NAME | Salary |
1 | Anna | 10000 |
2 | Maria | 20000 |
3 | Thomas | 30000 |
Read:
           Read means retrieving or fetching data or information from the table. We will use SELECT command to fetch data from the table. There is also an option of retrieving only those records which satisfy a particular condition by using the WHERE clause in a SELECT query.
SQL command to fetch data:
SELECT *from employee;
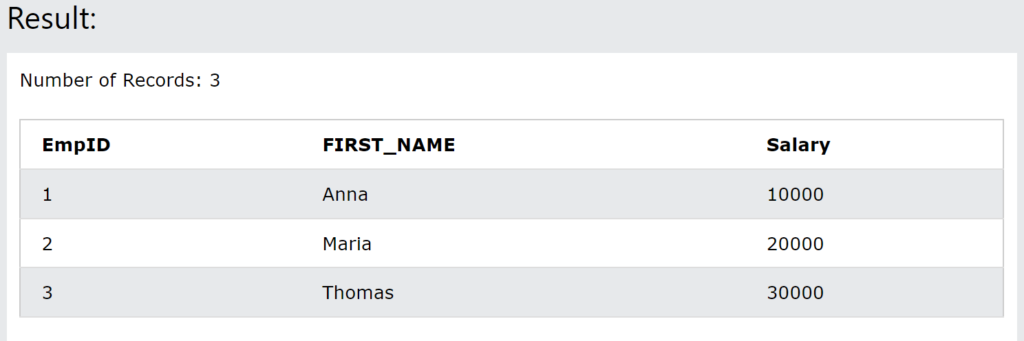
Update:
           Update operation makes changes in the table by modifying the data. We will use UPDATE command to make changes in the data present in tables.
SQL command to Update records:
Update employee SET EmpID = 4 WHERE First_Name = ‘Thomas’;
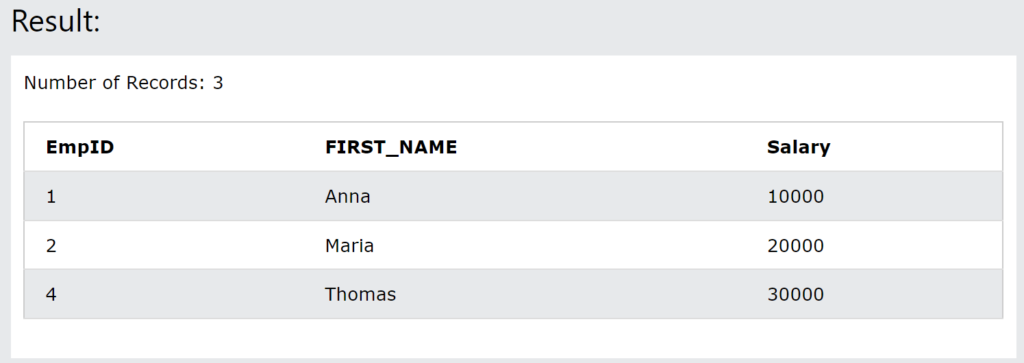
Delete:
Delete operation deletes the records from table. We can delete all the rows or a particular row using DELETE query.
SQL command to delete records:
DELETE FROM employee where Salary = 10000;
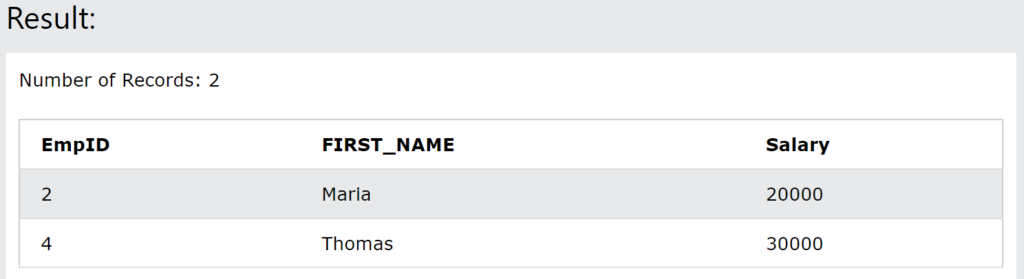
These are the basic CRUD operations in SQL. We also have another article about CRUD operation in Python. If you are interested you can go and check the article.