In this blog, we are going to see a sample program to handle CRUD operations on mysql DB from python interface. CRUD operation stands for CREATE, READ, UPDATE and DELETE. Here we are going to using Object oriented approach to better understand the functionality.
Pre – Requisite:
- Connection to MYSQL Database
- Database Plugin
- Mysql Python connector packages
We have already posted a blog on this prerequisite. Please feel free to have a look on the same.
Let’s understand the CRUD Functions
CREATE Function:
In this example we are going to create a table named Nutrients with 3 columns say ID, NAME and PERCENTAGE.
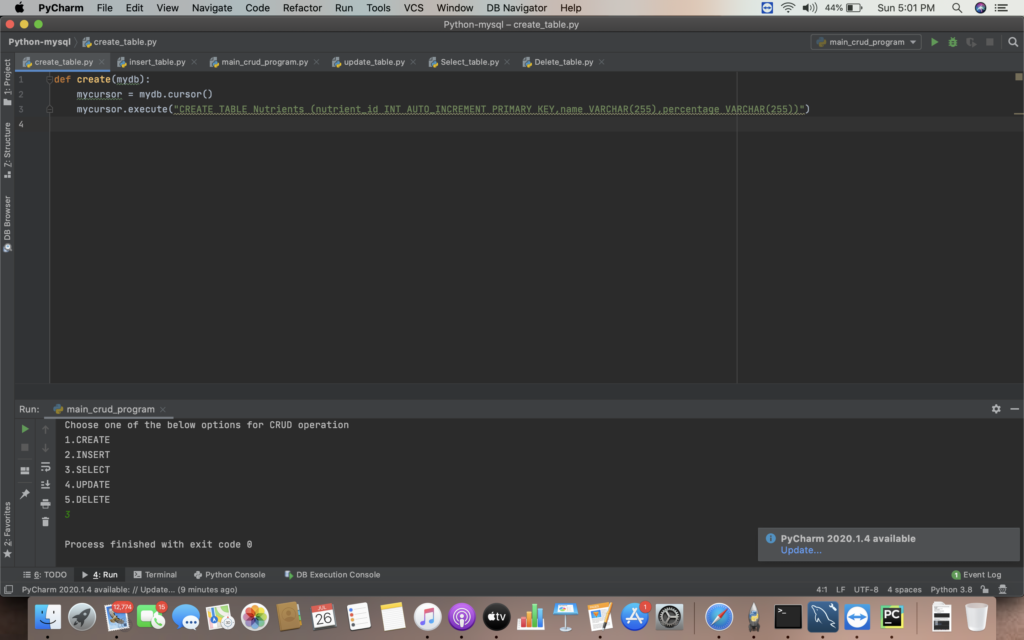
INSERT Function:
In the insert method we will get the user input values for the table and execute insert query into the Nutrients table.
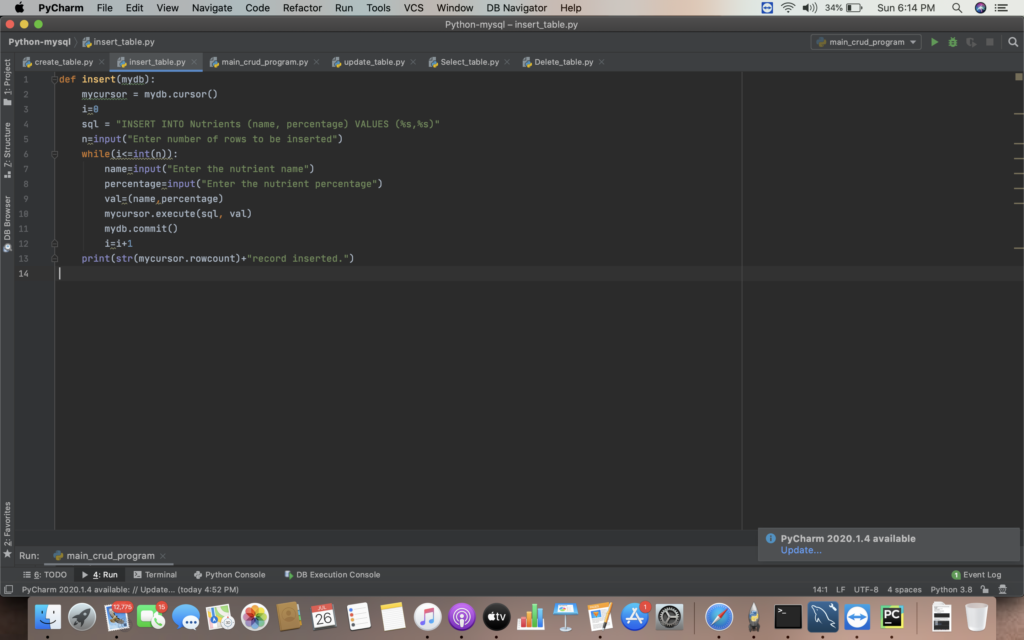
READ Function (SELECT Statement):
In the read (select) python method, the values stored in the Nutrients table will be displayed.
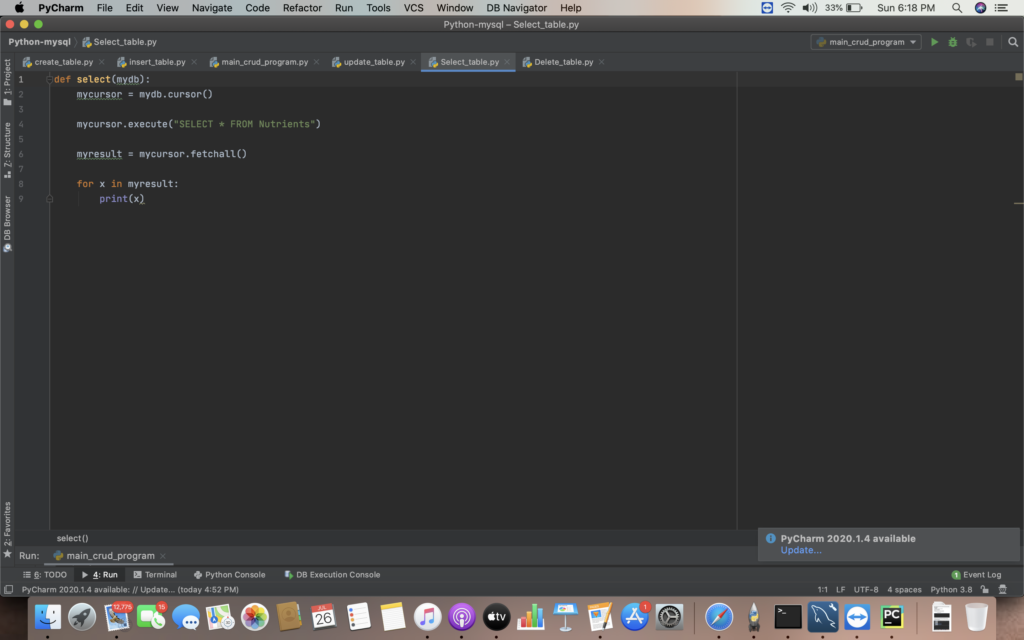
UPDATE Function:
In the update python method , update statements on Nutrient table will be executed based on the Where clause conditions.
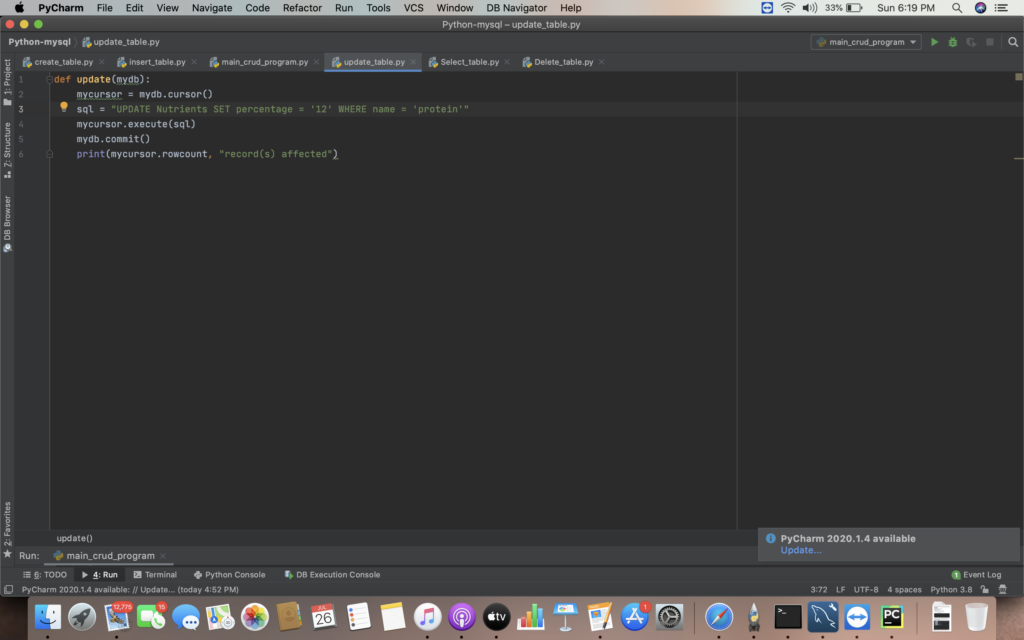
DELETE Function:
Delete method depicts how the records are deleted from the Nutrients table based on the conditions.
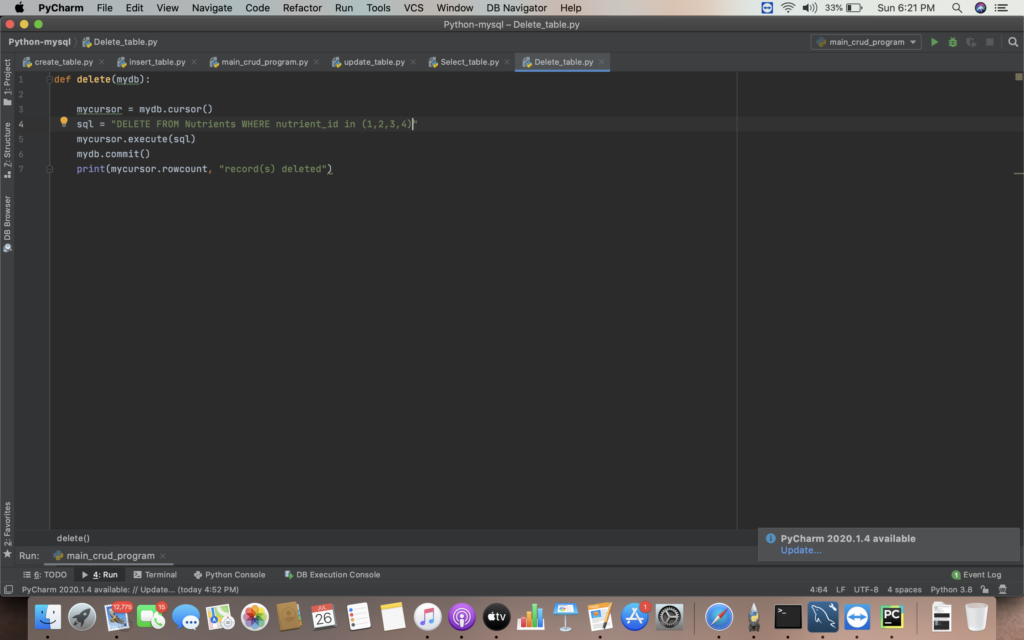
MAIN Program:
Main program file in python defines a user defined class called “Crud” .
It has two methods 1) InputChoices 2) crudfunctions.
InputChoices methods get’s user choice on which CRUD operation to be performed on the NUTRIENTS table
Crudfunctions method calls the corresponding python package and method for the user’s input choice.
C1 is the object instantiated for the Crud() class.
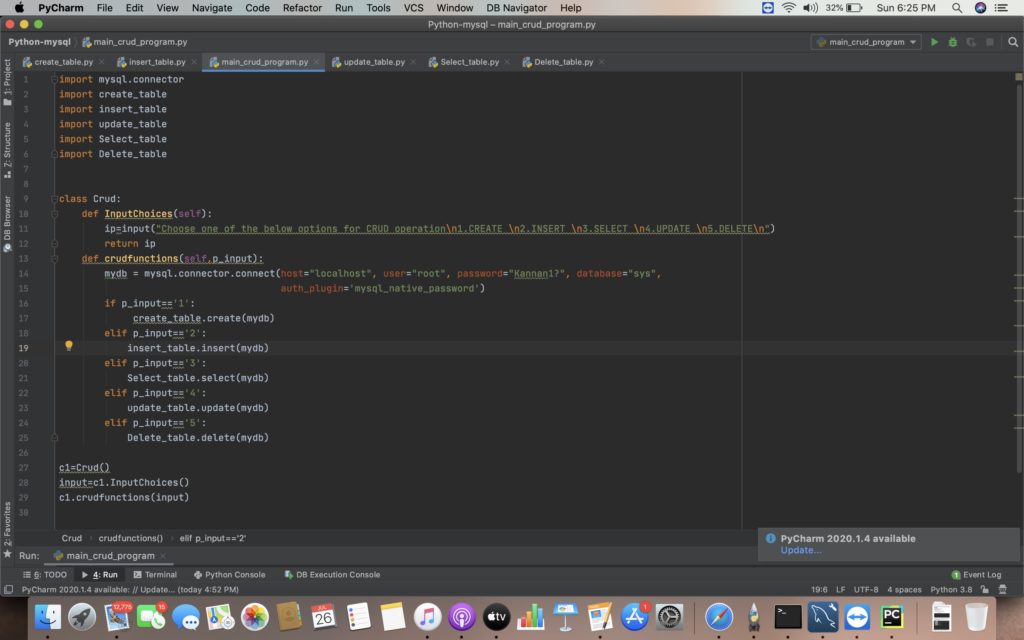
Execution of Program
i)Enter the choice 1 to create the table NUTRIENTS.
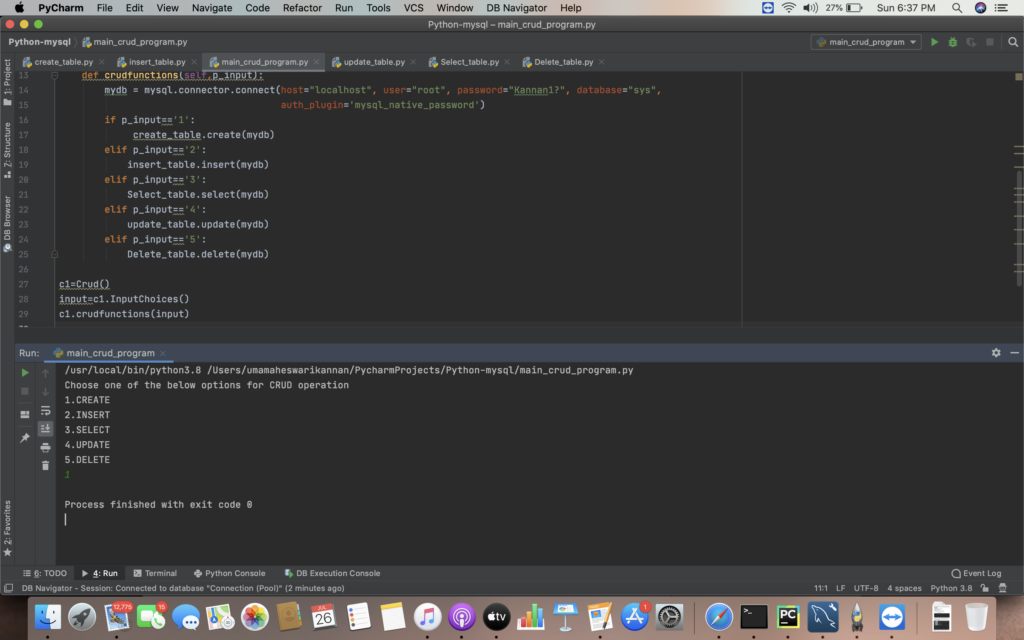
ii) Enter the choice 2 to perform insert into NUTRIENTS Table and give the corresponding values to be inserted.
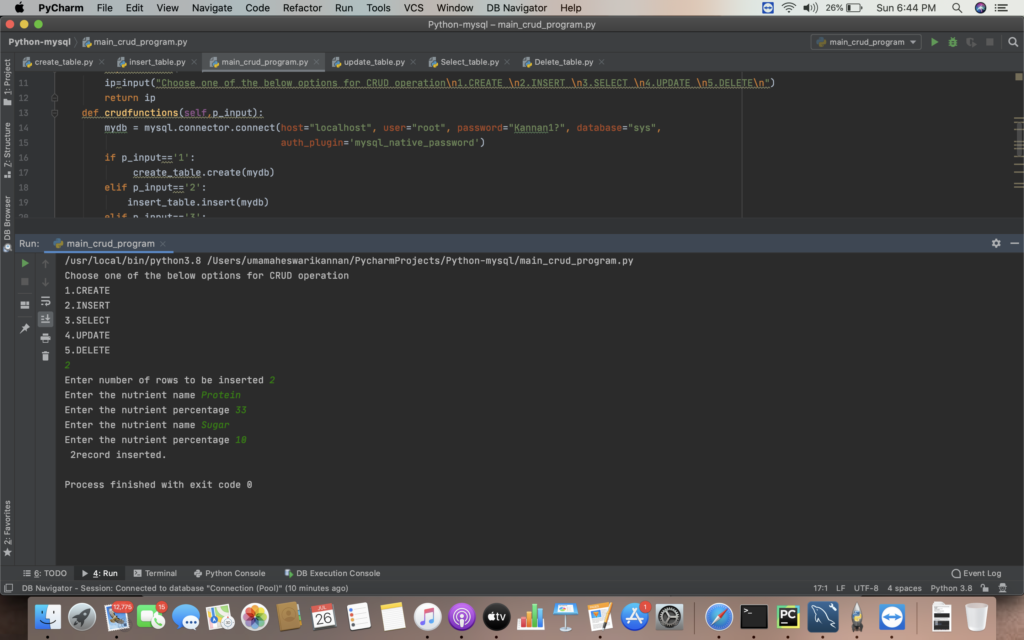
iii) Enter choice 3 to read the records from the table
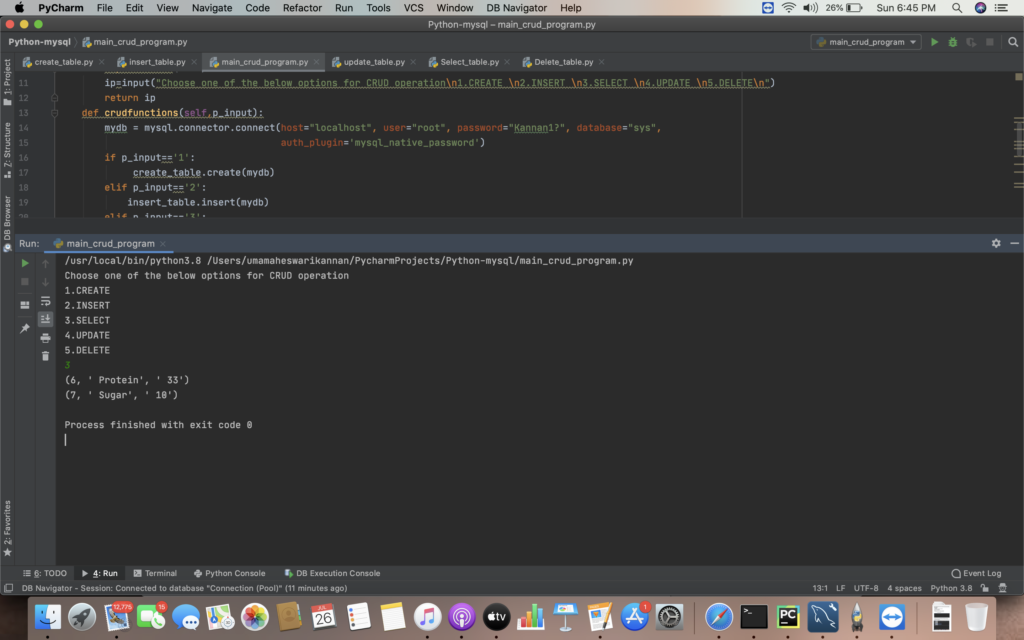
iv) Enter choice 4 to update any of the values in the Table.
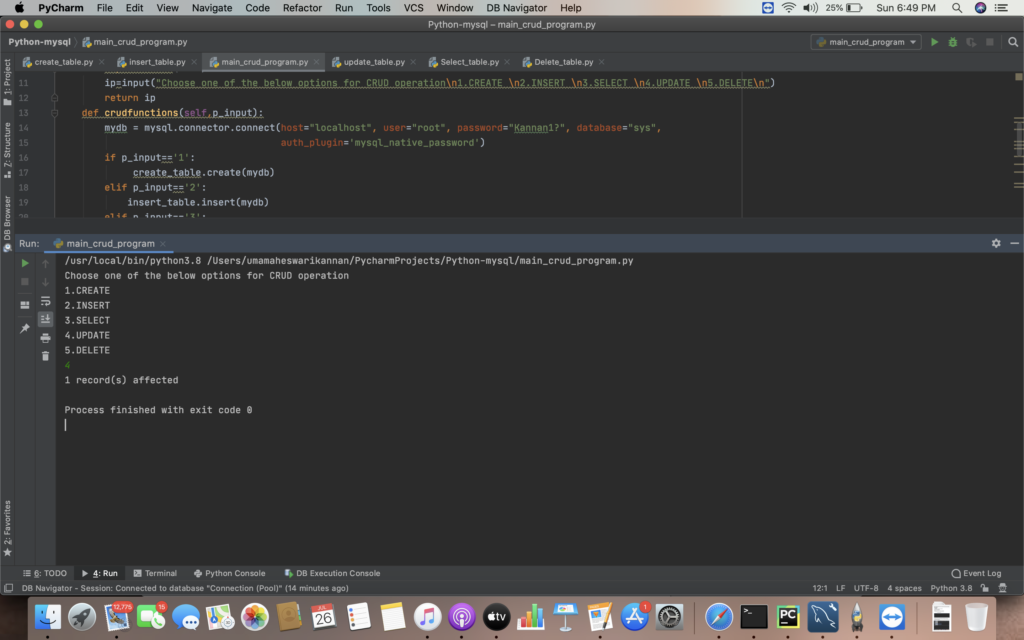
v) Enter choice 5 to delete records from the Table based on the conditions.
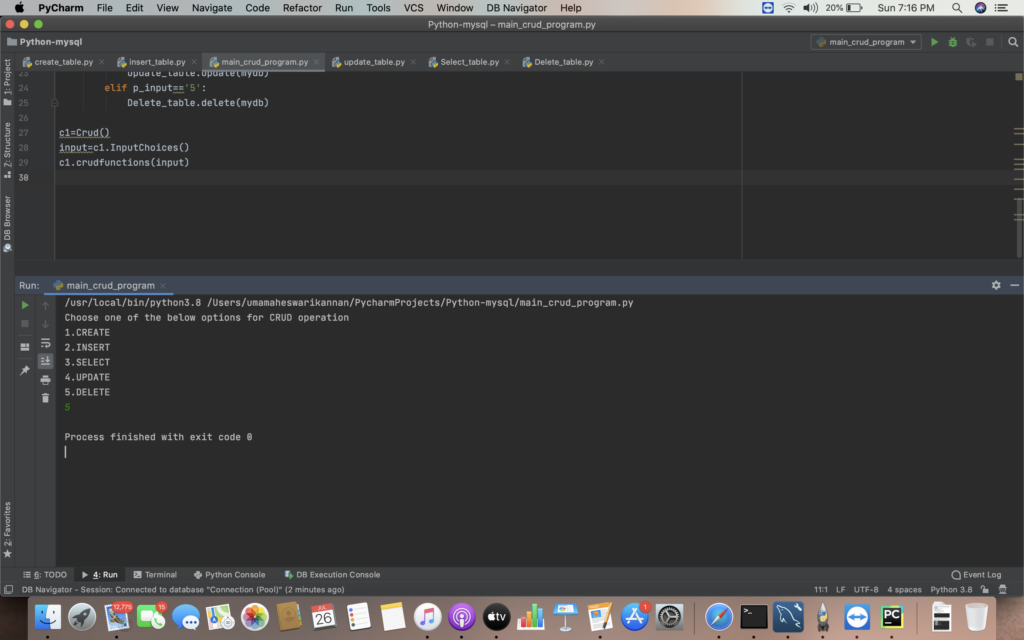
After deletion use the read function to check if delete has been performed.
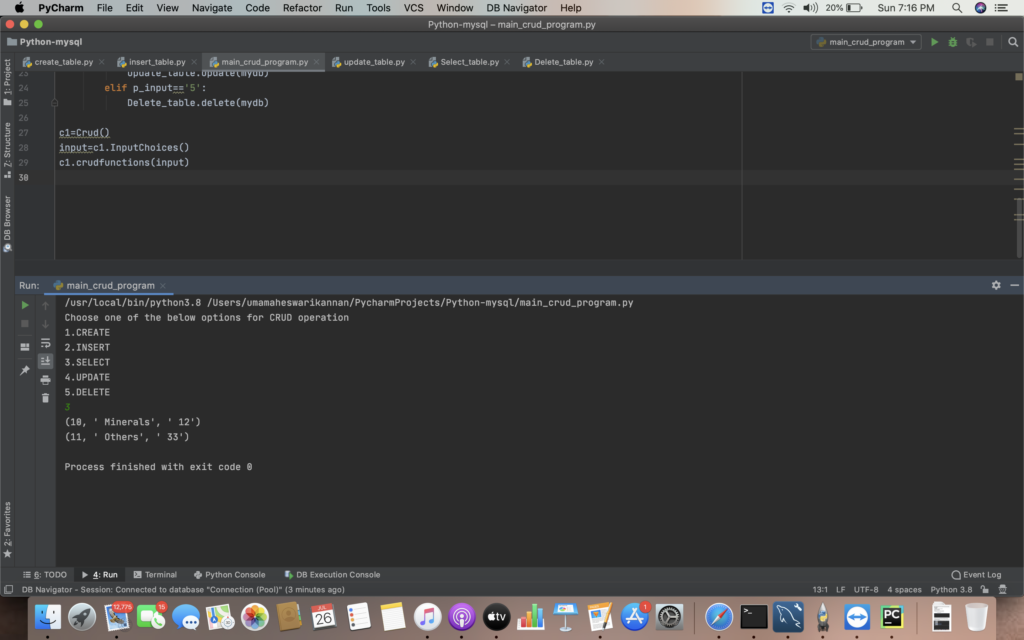