Map, reduce and filter are paradigms of functional programming. These helps in writing simple, short codes without worrying about iterations like loops and branching.
Functional Programming:
A Programming paradigm that uses functions to define a computation is known as functional programming. One important feature is concept of unchangeable state. This works only on immutable data types, we only work on copies of original data structure. Map, reduce and filter in python create and work with copy of data set leaving the original data set intact.
Higher – Order Functions:
These take functions as parameter and returns functions as result. Map(), reduce() and filter() are three most useful higher-order functions in Python. They can do complex operations when combined with simpler functions.
Map and filter are built-in functions and doesn’t require importing. Reduce resides in functools module so requires importing.
map():
map() function returns a map object ( which is an iterator or generator object) of the results after applying the given function to each item of a given iterable (list, tuple…,)
Syntax:
map (func, *iterables)
func: It is a function to which map passes each element of given iterable.
Iterables: It is to be mapped.
You can pass one or more iterables to the map function.
It returns the list of results after applying the given function to each of the given iterables ( list, tuples, hash tables etc.,)
The returned value from map() (map object) then can be passed to list ( to create a list), set ( to create a set).
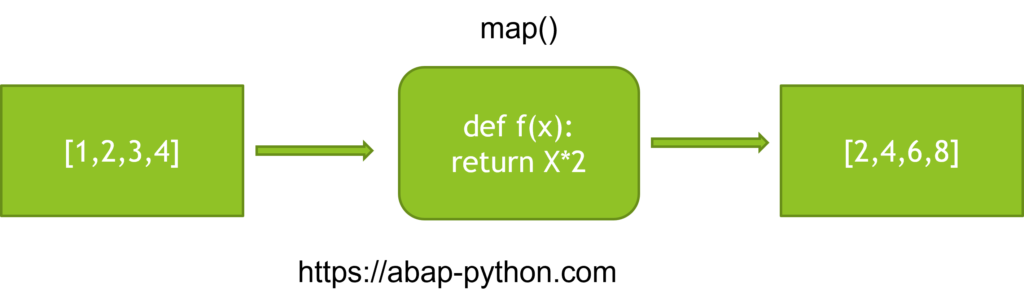
Example:
Using lambda expression for the above code.
Adding two lists using map and lambda:
filter():
filter() method filters the given sequence with the help of a function that tests each element in the sequence to be true or not.
Syntax:
filter(func, iterables)
Parameters:
function: function that tests if each element of a
sequence true or not.
sequence: sequence which needs to be filtered, it can be sets, lists, tuples, or containers of any iterators.
Returns:
returns an iterator that is already filtered.
Example:
Using lambda expression:
reduce():
reduce() function applies the provided function to the iterables and returns single value as result.
Syntax:
reduce(function, iterables)
The function specifies which expression should be applied to the ‘iterables’ in this case. The function tools module must be used to import this function.
Example:
Using lambda expression:
These are the most powerful higher order functions in python.