Steps involved in determining the total line items of a sap.m.Table
- get the sap.m.Table instance
- call the getItems() method of the sap.m.Table instance
- Loop through the records. For each row, get the mAggregations of the line item and then get the text property by accessing the cells of the item.
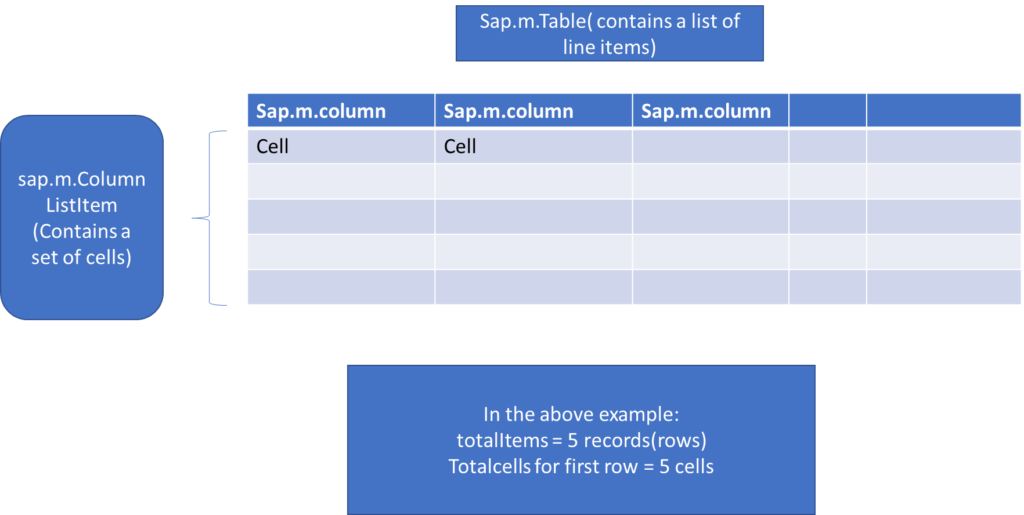
Main Code Below:
var tableItems = mTable.getItems();
document.write("Total no of items:", tableItems.length);
for(iRow = 0; iRow < tableItems.length; iRow++)
{
document.write("<br>");
document.write(tableItems[iRow].mAggregations.cells[0].mProperties.text);
document.write(",",tableItems[iRow].mAggregations.cells[1].mProperties.text)
document.write(",",tableItems[iRow].mAggregations.cells[2].mProperties.text);
}
Full code: Please run it in https://jsbin.com/?html,output
<!DOCTYPE html>
<html>
<head>
<meta name="description" content="Sap m Table">
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>SAP M TABLE</title>
<script id='sap-ui-bootstrap' type='text/javascript'
src='https://sapui5.hana.ondemand.com/resources/sap-ui-core.js'
data-sap-ui-theme='sap_bluecrystal'
data-sap-ui-libs='sap.m,sap.ui.table'
data-sap-ui-compatVersion='edge'></script>
<script type='text/javascript'>
var jsonData = [ {columnOne : "Data row A1", columnTwo : "Data row 100", columnThree : "Data row hundred"},
{columnOne : "Data row A2", columnTwo : "Data row 200", columnThree : "Data row two hundred"},
{columnOne : "Data row A3", columnTwo : "Data row 300", columnThree : "Data row three hundred"} ];
var jsonModel = new sap.ui.model.json.JSONModel(jsonData);
var mTable = new sap.m.Table({
columns : [
new sap.m.Column({ header : new sap.m.Label({ text : "Column One" }) }),
new sap.m.Column({ header : new sap.m.Label({ text : "Column Two" }) }),
new sap.m.Column({ header : new sap.m.Label({ text : "Column Three" }) })
]
}).placeAt("content");
mTable.setModel(jsonModel);
mTable.bindAggregation("items", {
path : "/",
template : new sap.m.ColumnListItem({
cells : [
new sap.m.Text({ text : "{columnOne}" }),
new sap.m.Text({ text : "{columnTwo}" }),
new sap.m.Text({ text : "{columnThree}" }),
]
})
});
var tableItems = mTable.getItems();
document.write("Total no of items:", tableItems.length);
for(iRow = 0; iRow < tableItems.length; iRow++)
{
document.write("<br>");
document.write(tableItems[iRow].mAggregations.cells[0].mProperties.text);
document.write(",",tableItems[iRow].mAggregations.cells[1].mProperties.text)
document.write(",",tableItems[iRow].mAggregations.cells[2].mProperties.text);
}
</script>
</head>
<body class='sapUiBody'>
<div id='content'></div>
</body>
</html>