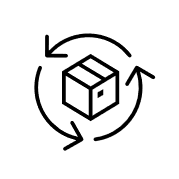
Generally, in programming languages loops are useful in repeating a block of code. Loops are repeated set of actions which runs based on a condition. The loop continues to run until the condition returns a FALSE. The basic idea of loop is to save time and effort. To loop in javascript we have 7 statements which belong to two categories.
There are mainly two types of loops:
- Entry controlled loop:
The condition is tested before entering the loop and the control is transferred into the loop only if the condition is TRUE. Example: for and while loops.
- Exit controlled loop:
The condition is tested at the end of the loop. The loop is executed at least once irrespective of whether the condition is TRUE or FALSE. Example: do-while loop.
1. for loop:
The most frequently used loop in javascript.
Syntax:
for(initialization; condition; iterator)
{
// code to execute
}
It consists of three parts. First part initialization is executed only once at the start of the loop and initializes the loop. The second part is a condition when it is evaluated to TRUE, the loop gets executed and terminates when it is FALSE. The third part is an iterator which usually a increments or decrements after each iteration. For loop is more readable as all the expressions lie on a single line. If the number of iterations is known we use for loop.
Example:
var sum = 0;
for (var i = 1; i <= 10; i++) {
sum = sum + i;
}
console.log("Sum = " + sum);
Output:
Sum = 55
2. for-in loop:
for-in loop iterates over the properties of an object. For each property the code in the block is executed. It iterates over the keys of an object which have their enumerable property to TRUE. It iterates over all the enumerable properties including inherited enumerable properties.
Syntax:
for( property in object){
//code
}
Example:
var employee = {name: "Tom", age: 25, salary: 1000};
for (var item in employee) {
console.log(employee[item]);
}
Output:
Tom
25
1000
3. for.. of loop:
for… of loop is used for iterating over iterable objects like arrays and strings.
Syntax:
for(variable of object){
//code
}
It can be used for iterating over arrays, strings, maps and set.
Example:
var employee=['Tom','Anna','Tim','Jones']
for (let item of employee)
{
console.log(item);
}
Output:
Tom
Anna
Tim
Jones
With Map:
const emp = new Map();
emp.set(1, "Tom");
emp.set(2, "Anna");
for (let i of emp) {
console.log(i);
}
Output:
Tom
Anna
With Set:
const emp = new Set();
emp.add(1);
emp.add("Tom");
for (let i of emp) {
console.log(i);
}
Output:
1
Tom
4. While Statement:
While loop starts by evaluating the condition. If it is TRUE the loop gets executed. If it is FALSE the loop terminates.
Syntax:
While(condition)
{
//statement
}
Example:
var sum = 0;
var number = 1;
while (number <= 10) { //condition
sum += number;
number++; //iterator
}
console.log("Sum = " + sum);
Output:
55
5.do… while statement:
Here the condition is checked at the end of the loop. The loop is executed atleast once irrespective of condition being TRUE or FALSE.
Syntax:
do {
// statement
} while (condition);
Example:
var sum = 0;
var number = 1;
do{
sum += number;
number++; //iterator
}while (number <= 10) //condition
console.log("Sum = " + sum);
Output:
55
6. break statement:
Whenever a break statement is seen in the loop, it exits the loop without executing other statements in the loop. Control is transferred to the statement following the loop.
Syntax:
for(initialization; condition; iterator){
// code
If(condition){
break;
}
//code
}
Example:
for(i=1;i<10;i++){
if(i==5){//break statement
break;
}
console.log(i);
}
Output:
1
2
3
4
7.continue statement:
continue statement is used to break the current iteration of the loop and the control flow of the program goes to the next iteration.
Syntax:
for(initialization; condition; iterator){
//code
if(condition){
continue;
}
//code
}
Example:
for(i=1;i<10;i++){
if(i==5){//continue statement
continue;
}
console.log(i);
}
Output:
1
2
3
4
6
7
8
9