Tkinter is a python package that provides various controls, such as buttons, labels, radio buttons, text area and text boxes used in a GUI application. These controls are commonly called widgets. The Button widget is used to display buttons in your application.
Lets learn these UI controls via a simple form creation program:
from tkinter import *
from tkinter import ttk
from tkinter import messagebox
window = Tk()
window.title("Contact Details")
window.geometry('1000x1000')
window.configure(background = "pink");
a = Label(window ,text = "Contact ID").grid(row = 0,column = 0)
b = Label(window ,text = "Contact Name").grid(row = 2,column = 0)
c = Label(window ,text = "Contact Address").grid(row = 4,column = 0)
d = Label(window ,text = "State").grid(row = 6,column = 0)
e = Label(window ,text = "City").grid(row = 8,column = 0)
f = Label(window ,text = "Mobile Number").grid(row = 10,column = 0)
g = Label(window ,text = "Gender").grid(row = 12,column = 0)
h = Label(window ,text = "Age").grid(row = 14,column = 0)
v=IntVar()
v.set(1)
r1=Radiobutton(window, text="male", variable=v,value=1).grid(row = 12,column = 1)
r2=Radiobutton(window, text="female", variable=v,value=2).grid(row = 12,column = 2)
list_of_state=[ 'Texas' ,'Arizona' , 'Illinois' ,'New Jersey' ,'Utah']
list_of_city=[ 'Houston' ,'Phoenix' ,'Chicago' , 'Atlantic City', 'Blanding']
#the variable 'c' mentioned here holds String Value, by default ""
c=StringVar()
droplist=OptionMenu(window,c, *list_of_state)
droplist.config(width=25)
c.set('Select your State')
droplist.grid(row = 6,column = 1)
#the variable 'c' mentioned here holds String Value, by default ""
c1=StringVar()
droplist1=OptionMenu(window,c1, *list_of_city)
droplist1.config(width=25)
c1.set('Select your City')
droplist1.grid(row = 8,column = 1)
a1 = Entry(window).grid(row = 0,column = 1)
b1 = Entry(window).grid(row = 2,column = 1)
c1 = Entry(window).grid(row = 4,column = 1)
d1 = Entry(window).grid(row = 10,column = 1)
f1 = Entry(window).grid(row = 14,column = 1)
def result():
messagebox.showinfo("information","Contact Details Saved Successfully")
btn = ttk.Button(window ,text="Submit",command=result).grid(row=40,column=3)
window.mainloop()
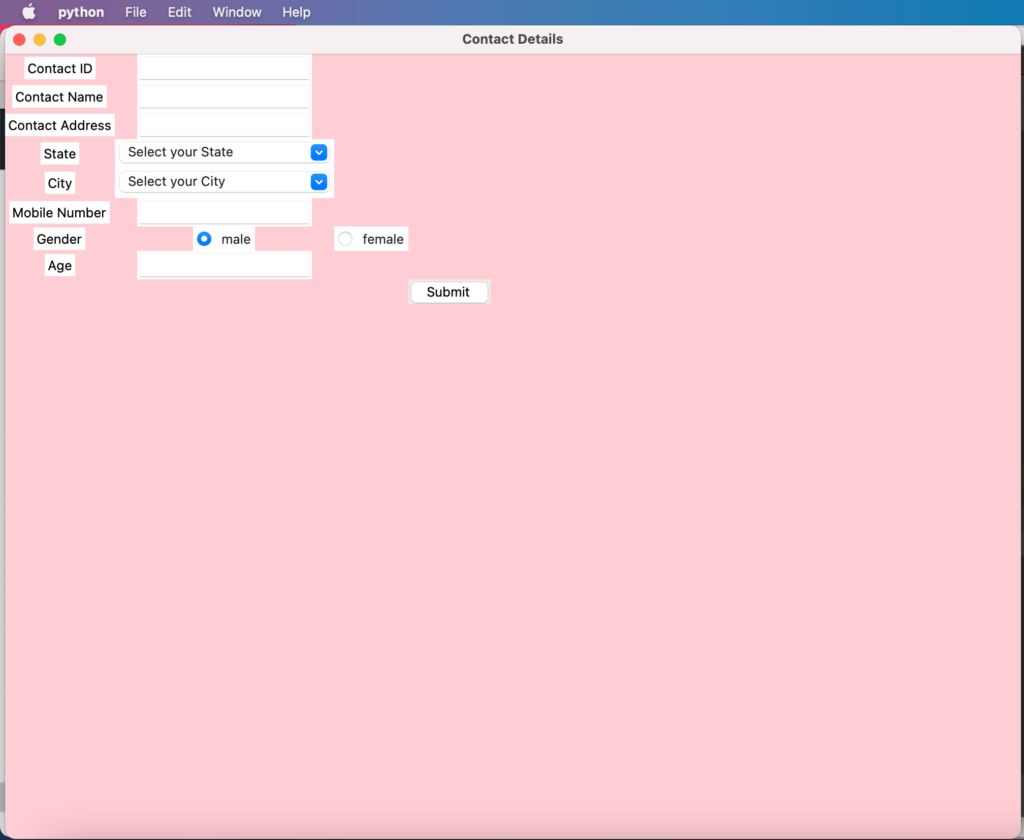