In this article, you will learn about the Type conversion and type casting in Python. Before learning type conversion in python, you should have knowledge about Python data types. To know about the data structures in python click here!
Type Conversion:
Python is a dynamically typed language, which means that we do not have to specify the data type of a variable we are assigning. The Python interpreter automatically understand the type of variables and assign data types accordingly. Type conversion is the process of converting one data type to another. It can be any data type like integer, float, string, list or dictionary. There are two types of type conversion
- Implicit Type conversion.
- Explicit Type conversion(Type casting).
Implicit Type Conversion:
In implicit type conversion, the python interpreter itself automatically converts the data type of a variable from one type to another without intervention of the user. Now let us see with an example code snippet how python interpreter converts a lower data type (int) to higher data type (float) to prevent data loss. This type of conversion is called upcasting.
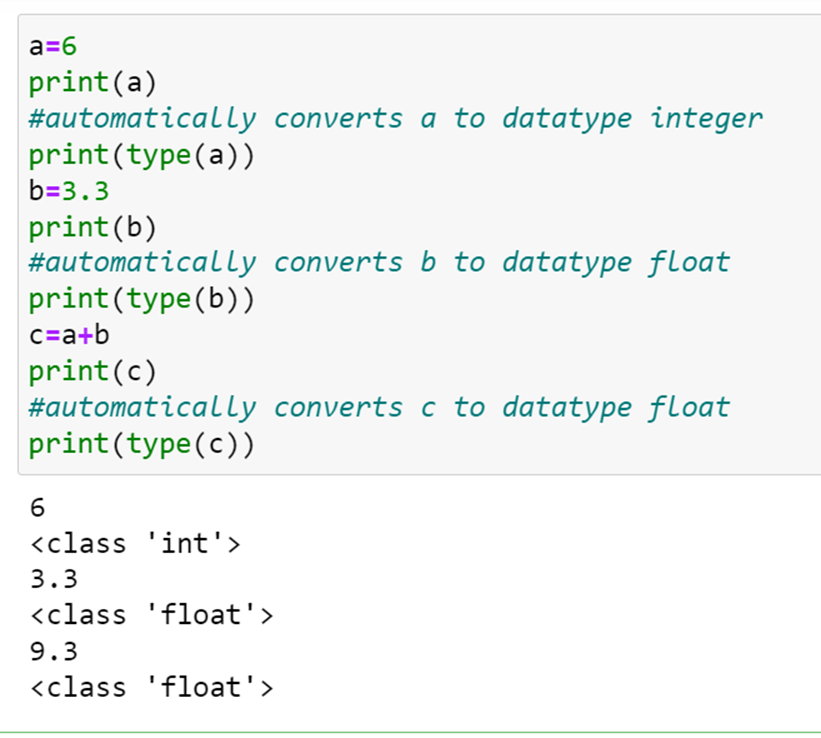
Now a question may arise what will happen if we try to add an integer and a string with + operator then we will get Type error. This is illustrated in the following code snippet.
However, we have a solution for this in explicit type conversion
Explicit Type conversion (Type casting):
In this method, python needs user involvement for converting one data type to another. The predefined functions in python to perform explicit type conversion are int(), float(), str(), bool().
Syntax:
(Required datatype) (Expression)
Now we will see some in-built Python functions and their descriptions
Function | Description |
Int(x[base]) | It converts x to an integer, and Base specifies the number base. For example, if you want to convert the string in decimal numbers then you’ll use 10 as base. |
Float(x) | It converts x to a floating-point number |
Str(x) | Converts x to string |
Tuple(x) | Converts x to tuple |
List(x) | Converts x to list |
Set(x) | Converts x to set |
Key Points to remember in Type conversion:
- Type conversion is the conversion of objects from one datatype to another.
- Implicit conversion is automatically performed by Python interpreter without loss of data.
- Explicit conversion also called as type casting is performed by involvement of user and data loss may occur as we enforce object to a specific data type.
These are all the things we need to note in type conversion. Most of the time implicit conversion will do its job. If we still need to convert to another datatype then explicit conversion is used.